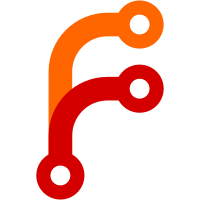
- Fixed bug in stream health function causing loop if track not active - Fixed DTSC pulls ignoring data before the live point - Improved async buffers (deque mode) to spread the tracks more fairly - DTSC pull now implements "ping" and "error" commands - DTSC pulls report suspicious keyframe intervals to the origin and ask for confirmation - DTSC output now accepts these reports and disconnects if there is no match in keyframe intervals - Outputs in async mode now keep the seek point in all tracks when reselecting - Outputs in async mode now default to a starting position in each track that is at a keyframe roughly halfway in the buffer - Outputs in async mode now ignore playback rate (always fastest possible) - Removed code duplication in prepareNext function - Reordered the prepareNext function somewhat to be easier to follow for humans - DTSC output no longer overrides initialSeek function, now uses default implementation - Sanitycheck output now supports both sync and async modes, supports printing multiple timestamps for multiple tracks
30 lines
814 B
C++
30 lines
814 B
C++
#include "output.h"
|
|
#include <mist/url.h>
|
|
|
|
namespace Mist{
|
|
|
|
class OutDTSC : public Output{
|
|
public:
|
|
OutDTSC(Socket::Connection &conn);
|
|
~OutDTSC();
|
|
static void init(Util::Config *cfg);
|
|
void onRequest();
|
|
void sendNext();
|
|
void sendHeader();
|
|
static bool listenMode(){return !(config->getString("target").size());}
|
|
void onFail(const std::string &msg, bool critical = false);
|
|
void stats(bool force = false);
|
|
void sendCmd(const JSON::Value &data);
|
|
void sendOk(const std::string &msg);
|
|
|
|
private:
|
|
unsigned int lastActive; ///< Time of last sending of data.
|
|
std::string getStatsName();
|
|
std::string salt;
|
|
HTTP::URL pushUrl;
|
|
void handlePush(DTSC::Scan &dScan);
|
|
void handlePlay(DTSC::Scan &dScan);
|
|
};
|
|
}// namespace Mist
|
|
|
|
typedef Mist::OutDTSC mistOut;
|