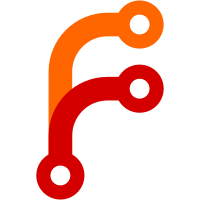
All processes:
- Added process status system and relevant API calls
- Added ability to set track masks for input/output in processes
- Added support for unmasking tracks when there is a push target, by the "unmask" parameter.
- Added track unmasking support for processes on exit/error
- Make processes start faster, if possible, in the first few seconds of a stream
- Delay stream ready state if there are processes attempting to start
Livepeer process updates:
- Added Content-Resolution header to MistProcLivepeer as per Livepeer's request
- Renamed transcode from "Mist Transcode" to source stream name
- Added ability to send audio to livepeer
- Robustified livepeer timing code, shutdown code, and improved GUI
- Prevent "audio keyframes" from starting segments in MistProcLivepeer
- Multithreaded (2 upload threads) livepeer process
- Stricter downloader/uploader timeout behaviour
- Robustness improvements
- Fix small segment size 😒
- Streamname correction
- Prevent getting stuck when transcoding multiple qualities and they are not equal length
- Corrected log message print error
- Race condition fix
- Now always waits for at least 1 video track
21 lines
882 B
C++
21 lines
882 B
C++
#include <mist/json.h>
|
|
|
|
namespace Controller{
|
|
void setProcStatus(uint64_t id, const std::string & proc, const std::string & source, const std::string & sink, const JSON::Value & status);
|
|
void getProcsForStream(const std::string & stream, JSON::Value & returnedProcList);
|
|
void procLogMessage(uint64_t id, const JSON::Value & msg);
|
|
bool isProcActive(uint64_t id);
|
|
bool streamsEqual(JSON::Value &one, JSON::Value &two);
|
|
void checkStream(std::string name, JSON::Value &data);
|
|
bool CheckAllStreams(JSON::Value &data);
|
|
void CheckStreams(JSON::Value &in, JSON::Value &out);
|
|
void AddStreams(JSON::Value &in, JSON::Value &out);
|
|
int deleteStream(const std::string &name, JSON::Value &out, bool sourceFileToo = false);
|
|
void checkParameters(JSON::Value &stream);
|
|
|
|
struct liveCheck{
|
|
long long int lastms;
|
|
long long int last_active;
|
|
};
|
|
|
|
}// namespace Controller
|