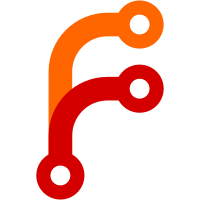
- Fixed segfault when attempting to initialseek on disconnected streams - Fix 100% CPU bug in controller's stats code - WebRTC UDP bind socket improvements - Several segfault fixes - Increased packet reordering buffer size from 30 to 150 packets - Tweaks to default output/buffer behaviour for incoming pushes - Added message for load balancer checks - Fixed HLS content type - Stats fixes - Exit reason fixes - Fixed socket IP address detection - Fixed non-string arguments for stream settings - Added caching for getConnectedBinHost() - Added WebRTC playback rate control - Added/completed VP8/VP9 support to WebRTC/RTSP - Added live seek option to WebRTC - Fixed seek to exactly newest timestamp - Fixed HLS input # Conflicts: # lib/defines.h # src/input/input.cpp
64 lines
1.8 KiB
C++
64 lines
1.8 KiB
C++
#include "input.h"
|
|
|
|
#include <set>
|
|
#include <stdio.h> //for FILE
|
|
|
|
#include <mist/dtsc.h>
|
|
|
|
namespace Mist{
|
|
///\brief A simple structure used for ordering byte seek positions.
|
|
struct seekPos{
|
|
///\brief Less-than comparison for seekPos structures.
|
|
///\param rhs The seekPos to compare with.
|
|
///\return Whether this object is smaller than rhs.
|
|
bool operator<(const seekPos &rhs) const{
|
|
if (seekTime < rhs.seekTime){return true;}
|
|
if (seekTime == rhs.seekTime){return trackID < rhs.trackID;}
|
|
return false;
|
|
}
|
|
uint64_t seekTime; ///< Stores the timestamp of the DTSC packet referenced by this structure.
|
|
uint64_t bytePos; ///< Stores the byteposition of the DTSC packet referenced by this structure.
|
|
uint32_t trackID; ///< Stores the track the DTSC packet referenced by this structure is
|
|
///< associated with.
|
|
};
|
|
|
|
class inputDTSC : public Input{
|
|
public:
|
|
inputDTSC(Util::Config *cfg);
|
|
bool needsLock();
|
|
|
|
virtual std::string getConnectedBinHost(){
|
|
if (srcConn){return srcConn.getBinHost();}
|
|
return Input::getConnectedBinHost();
|
|
}
|
|
|
|
protected:
|
|
// Private Functions
|
|
bool openStreamSource();
|
|
void closeStreamSource();
|
|
void parseStreamHeader();
|
|
bool checkArguments();
|
|
bool readHeader();
|
|
bool needHeader();
|
|
void getNext(size_t idx = INVALID_TRACK_ID);
|
|
void getNextFromStream(size_t idx = INVALID_TRACK_ID);
|
|
void seek(uint64_t seekTime, size_t idx = INVALID_TRACK_ID);
|
|
|
|
FILE *F;
|
|
|
|
Socket::Connection srcConn;
|
|
|
|
bool lockCache;
|
|
bool lockNeeded;
|
|
|
|
std::set<seekPos> currentPositions;
|
|
|
|
uint64_t lastreadpos;
|
|
|
|
char buffer[8];
|
|
|
|
void seekNext(uint64_t ms, size_t trackIdx, bool forceSeek = false);
|
|
};
|
|
}// namespace Mist
|
|
|
|
typedef Mist::inputDTSC mistIn;
|